|
6801
|
Programación / .NET (C#, VB.NET, ASP) / Re: Librería de Snippets !! (Compartan aquí sus snippets)
|
en: 21 Agosto 2014, 13:58 pm
|
Unos métodos de uso genérico para utilizar la librería IconLib ( http://www.codeproject.com/Articles/16178/IconLib-Icons-Unfolded-MultiIcon-and-Windows-Vista ) para crear iconos o leer las capas de un icono. PD: Hay que modificar un poco el source (escrito en C#) para permitir la creación de iconos de 512 x 512 (es facil, busquen un if con "256" y añadan el valor "512" a la enumeración de formatos de iconos), pero por otro lado no hay ningún problema para leer este tamaño de icono sin realizar modificaciones. 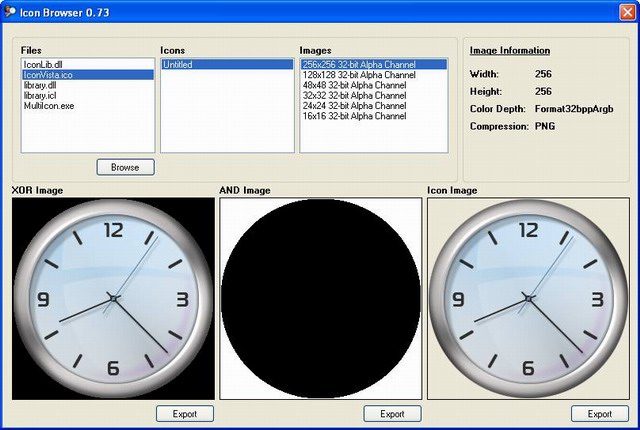 ' Create Icon ' By Elektro ' ' Usage Examples: ' ' Dim IconFile As IconLib.SingleIcon = CreateIcon("C:\Image.ico", IconLib.IconOutputFormat.All) ' For Each IconLayer As IconLib.IconImage In IconFile ' PictureBox1.BackgroundImage = IconLayer.Icon.ToBitmap ' Debug.WriteLine(IconLayer.Icon.Size.ToString) ' Application.DoEvents() ' Threading.Thread.Sleep(750) ' Next IconLayer ' ''' <summary> ''' Creates an icon with the specified image. ''' </summary> ''' <param name="imagefile">Indicates the image.</param> ''' <param name="format">Indicates the icon format.</param> ''' <returns>IconLib.SingleIcon.</returns> Public Function CreateIcon(ByVal imagefile As String, Optional ByVal format As IconLib.IconOutputFormat = IconLib.IconOutputFormat.All) As IconLib.SingleIcon Dim sIcon As IconLib.SingleIcon = New IconLib.MultiIcon().Add("Icon1") sIcon.CreateFrom(imagefile, format) Return sIcon End Function ' Get Icon-Layers ' By Elektro ' ' Usage Examples: ' ' For Each IconLayer As IconLib.IconImage In GetIconLayers("C:\Image.ico") ' PictureBox1.BackgroundImage = IconLayer.Icon.ToBitmap ' Debug.WriteLine(IconLayer.Icon.Size.ToString) ' Application.DoEvents() ' Threading.Thread.Sleep(750) ' Next IconLayer ' ''' <summary> ''' Gets all the icon layers inside an icon file. ''' </summary> ''' <param name="iconfile">Indicates the icon file.</param> ''' <returns>IconLib.SingleIcon.</returns> Public Function GetIconLayers(ByVal iconfile As String) As IconLib.SingleIcon Dim mIcon As IconLib.MultiIcon = New IconLib.MultiIcon() mIcon.Load(iconfile) Return mIcon.First End Function
|
|
|
6802
|
Programación / .NET (C#, VB.NET, ASP) / Re: Librería de Snippets !! (Compartan aquí sus snippets)
|
en: 21 Agosto 2014, 13:03 pm
|
Una class para ordenar los items de un listview según la columna:  ' *********************************************************************** ' Author : Elektro ' Last Modified On : 08-20-2014 ' *********************************************************************** ' <copyright file="ListView Column-Sorter.vb" company="Elektro Studios"> ' Copyright (c) Elektro Studios. All rights reserved. ' </copyright> ' *********************************************************************** #Region " Usage Examples " 'Public Class ListViewColumnSorter_TestForm : Inherits form ' ' ''' <summary> ' ''' The listview to sort. ' ''' </summary> ' Private WithEvents LV As New ListView ' ' ''' <summary> ' ''' The 'ListViewColumnSorter' instance. ' ''' </summary> ' Private Sorter As New ListViewColumnSorter ' ' ''' <summary> ' ''' Initializes a new instance of the <see cref="ListViewColumnSorter_TestForm"/> class. ' ''' </summary> ' Public Sub New() ' ' ' This call is required by the designer. ' InitializeComponent() ' ' With LV ' Set the Listview properties. ' ' ' Set the sorter, our 'ListViewColumnSorter'. ' .ListViewItemSorter = Sorter ' ' ' The sorting default direction. ' .Sorting = SortOrder.Ascending ' ' ' Set the default sort-modifier. ' Sorter.SortModifier = ListViewColumnSorter.SortModifiers.SortByText ' ' ' Add some columns. ' .Columns.Add("Text").Tag = ListViewColumnSorter.SortModifiers.SortByText ' .Columns.Add("Numbers").Tag = ListViewColumnSorter.SortModifiers.SortByNumber ' .Columns.Add("Dates").Tag = ListViewColumnSorter.SortModifiers.SortByDate ' ' ' Adjust the column sizes. ' For Each col As ColumnHeader In LV.Columns ' col.Width = 100I ' Next ' ' ' Add some items. ' .Items.Add("hello").SubItems.AddRange({"1", "11/11/2000"}) ' .Items.Add("yeehaa!").SubItems.AddRange({"2", "11-11-2000"}) ' .Items.Add("El3ktr0").SubItems.AddRange({"10", "9/9/1999"}) ' .Items.Add("wow").SubItems.AddRange({"100", "21/08/2014"}) ' ' ' Visual-Style things. ' .Dock = DockStyle.Fill ' .View = View.Details ' .FullRowSelect = True ' ' End With ' ' With Me ' Set the Form properties. ' ' .Size = New Size(400, 200) ' .FormBorderStyle = Windows.Forms.FormBorderStyle.FixedSingle ' .MaximizeBox = False ' .StartPosition = FormStartPosition.CenterScreen ' .Text = "ListViewColumnSorter TestForm" ' ' End With ' ' ' Add the Listview to UI. ' Me.Controls.Add(LV) ' ' End Sub ' ' ''' <summary> ' ''' Handles the 'ColumnClick' event of the 'ListView1' control. ' ''' </summary> ' Private Sub ListView1_ColumnClick(ByVal sender As Object, ByVal e As ColumnClickEventArgs) _ ' Handles LV.ColumnClick ' ' ' Dinamycaly sets the sort-modifier to sort the column by text, number, or date. ' Sorter.SortModifier = sender.columns(e.Column).tag ' ' ' Determine whether clicked column is already the column that is being sorted. ' If e.Column = Sorter.Column Then ' ' ' Reverse the current sort direction for this column. ' If Sorter.Order = SortOrder.Ascending Then ' Sorter.Order = SortOrder.Descending ' ' Else ' Sorter.Order = SortOrder.Ascending ' ' End If ' Sorter.Order ' ' Else ' ' ' Set the column number that is to be sorted, default to ascending. ' Sorter.Column = e.Column ' Sorter.Order = SortOrder.Ascending ' ' End If ' e.Column ' ' ' Perform the sort with these new sort options. ' sender.Sort() ' ' End Sub ' 'End Class #End Region #Region " Imports " Imports System.Text.RegularExpressions Imports System.ComponentModel #End Region #Region " ListView Column-Sorter " ''' <summary> ''' Performs a sorting comparison. ''' </summary> Public Class ListViewColumnSorter : Implements IComparer #Region " Objects " '''' <summary> '''' Indicates the comparer instance. '''' </summary> Private Comparer As Object = New TextComparer #End Region #Region " Properties " ''' <summary> ''' Gets or sets the number of the column to which to apply the sorting operation (Defaults to '0'). ''' </summary> Public Property Column As Integer Get Return Me._Column End Get Set(ByVal value As Integer) Me._Column = value End Set End Property Private _Column As Integer = 0I ''' <summary> ''' Gets or sets the order of sorting to apply. ''' </summary> Public Property Order As SortOrder Get Return Me._Order End Get Set(ByVal value As SortOrder) Me._Order = value End Set End Property Private _Order As SortOrder = SortOrder.None ''' <summary> ''' Gets or sets the sort modifier. ''' </summary> ''' <value>The sort modifier.</value> Public Property SortModifier As SortModifiers Get Return Me._SortModifier End Get Set(ByVal value As SortModifiers) Me._SortModifier = value End Set End Property Private _SortModifier As SortModifiers = SortModifiers.SortByText #End Region #Region " Enumerations " ''' <summary> ''' Specifies a comparison result. ''' </summary> Public Enum ComparerResult As Integer ''' <summary> ''' 'X' is equals to 'Y'. ''' </summary> Equals = 0I ''' <summary> ''' 'X' is less than 'Y'. ''' </summary> Less = -1I ''' <summary> ''' 'X' is greater than 'Y'. ''' </summary> Greater = 1I End Enum ''' <summary> ''' Indicates a Sorting Modifier. ''' </summary> Public Enum SortModifiers As Integer ''' <summary> ''' Treats the values ​​as text. ''' </summary> SortByText = 0I ''' <summary> ''' Treats the values ​​as numbers. ''' </summary> SortByNumber = 1I ''' <summary> ''' Treats valuesthe values ​​as dates. ''' </summary> SortByDate = 2I End Enum #End Region #Region " Private Methods " ''' <summary> ''' Compares two objects and returns a value indicating whether one is less than, equal to, or greater than the other. ''' </summary> ''' <param name="x">The first object to compare.</param> ''' <param name="y">The second object to compare.</param> ''' <returns> ''' A signed integer that indicates the relative values of <paramref name="x"/> and <paramref name="y"/>, ''' 0: <paramref name="x"/> equals <paramref name="y"/>. ''' Less than 0: <paramref name="x"/> is less than <paramref name="y"/>. ''' Greater than 0: <paramref name="x"/> is greater than <paramref name="y"/>. ''' </returns> Private Function Compare(ByVal x As Object, ByVal y As Object) As Integer Implements IComparer.Compare Dim CompareResult As ComparerResult = ComparerResult.Equals Dim LVItemX, LVItemY As ListViewItem ' Cast the objects to be compared LVItemX = DirectCast(x, ListViewItem) LVItemY = DirectCast(y, ListViewItem) Dim strX As String = If(Not LVItemX.SubItems.Count <= Me._Column, LVItemX.SubItems(Me._Column).Text, Nothing) Dim strY As String = If(Not LVItemY.SubItems.Count <= Me._Column, LVItemY.SubItems(Me._Column).Text, Nothing) Dim listViewMain As ListView = LVItemX.ListView ' Calculate correct return value based on object comparison If listViewMain.Sorting <> SortOrder.Ascending AndAlso listViewMain.Sorting <> SortOrder.Descending Then ' Return '0' to indicate they are equal Return ComparerResult.Equals End If If Me._SortModifier.Equals(SortModifiers.SortByText) Then ' Compare the two items If LVItemX.SubItems.Count <= Me._Column AndAlso LVItemY.SubItems.Count <= Me._Column Then CompareResult = Me.Comparer.Compare(Nothing, Nothing) ElseIf LVItemX.SubItems.Count <= Me._Column AndAlso LVItemY.SubItems.Count > Me._Column Then CompareResult = Me.Comparer.Compare(Nothing, strY) ElseIf LVItemX.SubItems.Count > Me._Column AndAlso LVItemY.SubItems.Count <= Me._Column Then CompareResult = Me.Comparer.Compare(strX, Nothing) Else CompareResult = Me.Comparer.Compare(strX, strY) End If Else ' Me._SortModifier IsNot 'SortByText' Select Case Me._SortModifier Case SortModifiers.SortByNumber If Me.Comparer.GetType <> GetType(NumericComparer) Then Me.Comparer = New NumericComparer End If Case SortModifiers.SortByDate If Me.Comparer.GetType <> GetType(DateComparer) Then Me.Comparer = New DateComparer End If Case Else If Me.Comparer.GetType <> GetType(TextComparer) Then Me.Comparer = New TextComparer End If End Select CompareResult = Comparer.Compare(strX, strY) End If ' Me._SortModifier.Equals(...) ' Calculate correct return value based on object comparison If Me._Order = SortOrder.Ascending Then ' Ascending sort is selected, return normal result of compare operation Return CompareResult ElseIf Me._Order = SortOrder.Descending Then ' Descending sort is selected, return negative result of compare operation Return (-CompareResult) Else ' Return '0' to indicate they are equal Return 0I End If ' Me._Order = ... End Function #End Region #Region " Hidden Methods " ''' <summary> ''' Serves as a hash function for a particular type. ''' </summary> <EditorBrowsable(EditorBrowsableState.Never)> Public Shadows Sub GetHashCode() End Sub ''' <summary> ''' Determines whether the specified System.Object instances are considered equal. ''' </summary> <EditorBrowsable(EditorBrowsableState.Never)> Public Shadows Sub Equals() End Sub ''' <summary> ''' Gets the System.Type of the current instance. ''' </summary> ''' <returns>The exact runtime type of the current instance.</returns> <EditorBrowsable(EditorBrowsableState.Never)> Public Shadows Function [GetType]() Return Me.GetType End Function ''' <summary> ''' Returns a String that represents the current object. ''' </summary> <EditorBrowsable(EditorBrowsableState.Never)> Public Shadows Sub ToString() End Sub #End Region End Class #End Region #Region " Comparers " #Region " Text " ''' <summary> ''' Performs a text comparison. ''' </summary> Public Class TextComparer : Inherits CaseInsensitiveComparer #Region " Enumerations " ''' <summary> ''' Specifies a comparison result. ''' </summary> Public Enum ComparerResult As Integer ''' <summary> ''' 'X' is equals to 'Y'. ''' </summary> Equals = 0I ''' <summary> ''' 'X' is less than 'Y'. ''' </summary> Less = -1I ''' <summary> ''' 'X' is greater than 'Y'. ''' </summary> Greater = 1I End Enum #End Region #Region " Methods " ''' <summary> ''' Compares two objects and returns a value indicating whether one is less than, equal to, or greater than the other. ''' </summary> ''' <param name="x">The first object to compare.</param> ''' <param name="y">The second object to compare.</param> ''' <returns> ''' A signed integer that indicates the relative values of <paramref name="x"/> and <paramref name="y"/>, ''' 0: <paramref name="x"/> equals <paramref name="y"/>. ''' Less than 0: <paramref name="x"/> is less than <paramref name="y"/>. ''' Greater than 0: <paramref name="x"/> is greater than <paramref name="y"/>. ''' </returns> Friend Shadows Function Compare(ByVal x As Object, ByVal y As Object) As Integer ' Null parsing. If x Is Nothing AndAlso y Is Nothing Then Return ComparerResult.Equals ' X is equals to Y. ElseIf x Is Nothing AndAlso y IsNot Nothing Then Return ComparerResult.Less ' X is less than Y. ElseIf x IsNot Nothing AndAlso y Is Nothing Then Return ComparerResult.Greater ' X is greater than Y. End If ' String parsing: If (TypeOf x Is String) AndAlso (TypeOf y Is String) Then ' True and True Return [Enum].Parse(GetType(ComparerResult), MyBase.Compare(x, y)) ElseIf (TypeOf x Is String) AndAlso Not (TypeOf y Is String) Then ' True and False Return ComparerResult.Greater ' X is greater than Y. ElseIf Not (TypeOf x Is String) AndAlso (TypeOf y Is String) Then ' False and True Return ComparerResult.Less ' X is less than Y. Else ' False and False Return ComparerResult.Equals End If End Function #End Region End Class #End Region #Region " Numeric " ''' <summary> ''' Performs a numeric comparison. ''' </summary> Public Class NumericComparer : Implements IComparer #Region " Enumerations " ''' <summary> ''' Specifies a comparison result. ''' </summary> Public Enum ComparerResult As Integer ''' <summary> ''' 'X' is equals to 'Y'. ''' </summary> Equals = 0I ''' <summary> ''' 'X' is less than 'Y'. ''' </summary> Less = -1I ''' <summary> ''' 'X' is greater than 'Y'. ''' </summary> Greater = 1I End Enum #End Region #Region " Methods " ''' <summary> ''' Compares two objects and returns a value indicating whether one is less than, equal to, or greater than the other. ''' </summary> ''' <param name="x">The first object to compare.</param> ''' <param name="y">The second object to compare.</param> ''' <returns> ''' A signed integer that indicates the relative values of <paramref name="x"/> and <paramref name="y"/>, ''' 0: <paramref name="x"/> equals <paramref name="y"/>. ''' Less than 0: <paramref name="x" /> is less than <paramref name="y"/>. ''' Greater than 0: <paramref name="x"/> is greater than <paramref name="y"/>. ''' </returns> Public Function Compare(ByVal x As Object, ByVal y As Object) As Integer _ Implements IComparer.Compare ' Null parsing. If x Is Nothing AndAlso y Is Nothing Then Return ComparerResult.Equals ' X is equals to Y. ElseIf x Is Nothing AndAlso y IsNot Nothing Then Return ComparerResult.Less ' X is less than Y. ElseIf x IsNot Nothing AndAlso y Is Nothing Then Return ComparerResult.Greater ' X is greater than Y. End If ' The single variables to parse the text. Dim SingleX, SingleY As Single ' Single parsing: If Single.TryParse(x, SingleX) AndAlso Single.TryParse(y, SingleY) Then ' True and True Return [Enum].Parse(GetType(ComparerResult), SingleX.CompareTo(SingleY)) ElseIf Single.TryParse(x, SingleX) AndAlso Not Single.TryParse(y, SingleY) Then ' True and False Return ComparerResult.Greater ' X is greater than Y. ElseIf Not Single.TryParse(x, SingleX) AndAlso Single.TryParse(y, SingleY) Then ' False and True Return ComparerResult.Less ' X is less than Y. Else ' False and False Return [Enum].Parse(GetType(ComparerResult), x.ToString.CompareTo(y.ToString)) End If End Function #End Region End Class #End Region #Region " Date " ''' <summary> ''' Performs a date comparison. ''' </summary> Public Class DateComparer : Implements IComparer #Region " Enumerations " ''' <summary> ''' Specifies a comparison result. ''' </summary> Public Enum ComparerResult As Integer ''' <summary> ''' 'X' is equals to 'Y'. ''' </summary> Equals = 0I ''' <summary> ''' 'X' is less than 'Y'. ''' </summary> Less = -1I ''' <summary> ''' 'X' is greater than 'Y'. ''' </summary> Greater = 1I End Enum #End Region #Region " Methods " ''' <summary> ''' Compares two objects and returns a value indicating whether one is less than, equal to, or greater than the other. ''' </summary> ''' <param name="x">The first object to compare.</param> ''' <param name="y">The second object to compare.</param> ''' <returns> ''' A signed integer that indicates the relative values of <paramref name="x"/> and <paramref name="y"/>, ''' 0: <paramref name="x"/> equals <paramref name="y"/>. ''' Less than 0: <paramref name="x"/> is less than <paramref name="y"/>. ''' Greater than 0: <paramref name="x"/> is greater than <paramref name="y"/>. ''' </returns> Public Function Compare(ByVal x As Object, ByVal y As Object) As Integer Implements IComparer.Compare ' Null parsing. If x Is Nothing AndAlso y Is Nothing Then Return ComparerResult.Equals ' X is equals to Y. ElseIf x Is Nothing AndAlso y IsNot Nothing Then Return ComparerResult.Less ' X is less than Y. ElseIf x IsNot Nothing AndAlso y Is Nothing Then Return ComparerResult.Greater ' X is greater than Y. End If ' The Date variables to parse the text. Dim DateX, DateY As Date ' Date parsing: If Date.TryParse(x, DateX) AndAlso Date.TryParse(y, DateY) Then ' True and True Return [Enum].Parse(GetType(ComparerResult), DateX.CompareTo(DateY)) ElseIf Date.TryParse(x, DateX) AndAlso Not Date.TryParse(y, DateY) Then ' True and False Return ComparerResult.Greater ' X is greater than Y. ElseIf Not Date.TryParse(x, DateX) AndAlso Date.TryParse(y, DateY) Then ' False and True Return ComparerResult.Less ' X is less than Y. Else ' False and False Return [Enum].Parse(GetType(ComparerResult), x.ToString.CompareTo(y.ToString)) End If End Function #End Region End Class #End Region #End Region
|
|
|
6803
|
Media / Multimedia / Re: Video .mp4 borroso
|
en: 21 Agosto 2014, 10:53 am
|
se puede ver claro pero en una ventana que rebasa la pantalla de mi monitor, y cuando trato de reducirlo un poco para adecuarla a la pantalla la imagen se distorsiona. ¿Así que a mayor resolución menor "distorsión"?, me parece que podría ser un problema de mala configuración del driver de salida que utilizas en el reproductor, o del renderizador que usas por defecto, o un problema de ambos. Mi pregunta antes de proseguir, ¿tienes instalado algún pack de codecs? (te puedes imaginar mi respuesta en caso de que si que tengas instalado alguno). Si dices que el VLC es el que menos te distoriona el video entonces ve a las opciones de la aplicación, y busca las configuraciones del driver de salida y del renderizador, aplica esa misma configuración a los reproductores que se te vean mal (en caso de que permitan configurar esos parámetros claro). Si en el reproductor que te funciona "mal" puedes configurar el driver de salida, escoje "Direct3D", y para el renderizador, prueba con " VMR9 (modo ventana)" o lee aquí ↠ Choosing the Right Video RendererSaludos!
|
|
|
6804
|
Programación / .NET (C#, VB.NET, ASP) / Re: Mis malas combinaciones :(
|
en: 21 Agosto 2014, 10:22 am
|
porque no me salen los números que he puesto para combinar ? Por esto: For Num As Integer = IndexCounter To (FixedValues.Count) Step NumStep ' 1 to 30 Step 3 Combo.Add(Num) ...
Estás tomando el número de la variable num en lugar de tomar un item de la colección FixedValues, se puede decir que esto ha sido un fallo mio porque como ya sabes en ese ejemplo usé una secuencia ordenada del 1 al 30, así que se me pasó por alto ese detalle al desordenar la secuencia xD. Prueba a ver si este es el resultado que quieres obtener: Combo.Add(FixedValues(NumStep * LenCounter)) Edito: o lo que viene a ser lo mismo:Combo.Add(FixedValues(Num - 1))
Explico: Para generar la primera combinación con los números que has mostrado, daría saltos de '3' para tomar estas posiciones: { 1, , , 22, , , 66, , , 20, } + un número aleatorio aleatorio del rango que especificaste EDITO: del rango de 'RandomValues', para dejarlo claro. Saludos
|
|
|
6806
|
Programación / .NET (C#, VB.NET, ASP) / Re: Información en int, hex y bin dentro de un Form
|
en: 21 Agosto 2014, 09:42 am
|
¿Cuál es la mejor forma de codearlo?[/b] Pues hombre... agregar 35 controles y usar 35 event-handlers para suscribirte al mismo evento desde luego que no es lo más eficiente, se puede reducir mucho el código para que sea siendo dinámico como dijo el compañero seba123neo, pero seguiría habiendo 35 controles en la UI, hay otras formas de hacerlo de forma dinámica (como también te explicó seba123neo). Mi consejo: 1. Utiliza solo 1 picturebox de fondo, y para todo lo demás utiliza las classes de GDI+. 2. Dibuja una rejilla del tamaño deseado sobre el picturebox, con las columnas y filas deseadas, en el evento Paint del picturebox. 3. Crea 1 Rectangle por cada sector de la rejilla, y así ya tienes una referencia de cada sector de la rejilla con su respectiva localización y tamaño a la que puedes acceder en cualquier momento. 4. En este punto ya se supone que deberías haber obtenido una coleción de los sectores de la rejilla( te sugiero añadirlos a una List(Of Rectangle) ), así que solo tienes que suscribirte a los eventos del Mouse ( MouseMove y MouseClick) del PictureBox donde harás las operaciones necesarias (como por ejemplo especificar en que sector de la rejilla se hizo click, y el color despues de hacer click, etc), fin del problema. Te muestro un ejemplo:  En ese ejemplo solo utilicé 1 picturebox con el fondo negro (aunque un picturebox tampoco es totalmente necesario pero facilita un poco la tarea), dibujé la rejilla con Pens, y para todo lo demás utilicé Rectangle, siguiendo los pasos que te he explicado.
No voy a mostrar todo el trabajo porque la idea es que aprendas a hacerlo pro ti mismo, y además, yo lo hice en VB.NET, peor te dejo una ayudita por si te sirve (lo puedes traducir a C# en convertidores online): Este método lo escribí para dibujar los márgenes del grid (lo debes utilizar en el evento Paint): ' Draw Grid ' By Elektro ' ''' <summary> ''' Draws a grid in the specified <see cref="System.Drawing.Graphics"/> space. ''' </summary> ''' <param name="g">Indicates the <see cref="System.Drawing.Graphics"/> object.</param> ''' <param name="GridSize">Indicates the size of the grid.</param> ''' <param name="Columns">Indicates the amount of columns to draw.</param> ''' <param name="Rows">Indicates the amount of rows to draw.</param> ''' <param name="ColorColumns">Indicates the columns color.</param> ''' <param name="ColorRows">Indicates the rows color.</param> ''' <param name="DrawBorder">If set to <c>true</c>, a border is drawn on the grid edges.</param> ''' <param name="ColorBorder">Indicates the border color. Default value is the same color as <param ref="ColorColumns"/></param> ''' <param name="ColorStyle">Indicates the colors <see cref="System.Drawing.Drawing2D.DashStyle"/>.</param> ''' <exception cref="System.ArgumentException"> ''' GridSize.Width is not divisible by the specified number of columns. ''' or ''' GridSize.Height is not divisible by the specified number of rows. ''' </exception> Private Sub DrawGrid(ByVal g As Graphics, ByVal GridSize As Size, ByVal Columns As Integer, ByVal Rows As Integer, ByVal ColorColumns As Color, ByVal ColorRows As Color, Optional ByVal DrawBorder As Boolean = True, Optional ByVal ColorBorder As Color = Nothing, Optional ByVal ColorStyle As Drawing2D.DashStyle = Drawing2D.DashStyle.Solid) If Not (GridSize.Width Mod Columns = 0I) Then Throw New ArgumentException( "GridSize.Width is not divisible by the specified number of columns.", "GridSize") Exit Sub ElseIf Not (GridSize.Height Mod Rows = 0I) Then Throw New ArgumentException( "GridSize.Height is not divisible by the specified number of rows.", "GridSize") Exit Sub End If Dim SectorWidth As Integer = (GridSize.Width \ Columns) Dim SectorHeight As Integer = (GridSize.Height \ Rows) Using PenRow As New Pen(ColorRows) With {.DashStyle = ColorStyle} Using PenCol As New Pen(ColorColumns) With {.DashStyle = ColorStyle} For row As Integer = 0I To GridSize.Height - 1 Step (SectorHeight) For col As Integer = 0I To GridSize.Width - 1 Step SectorWidth ' Draw the vertical grid-lines. g.DrawLine(PenCol, New Point(x:=col - 1, y:=0I), New Point(x:=col - 1, y:=GridSize.Height)) Next col ' Draw the horizontal grid-lines. g.DrawLine(PenRow, New Point(x:=0I, y:=row - 1), New Point(x:=GridSize.Width, y:=row - 1)) Next row If DrawBorder Then Using PenBorder As New Pen(If(ColorBorder = Nothing, ColorColumns, ColorBorder)) With {.DashStyle = ColorStyle} ' Draw the vertical left grid-line. g.DrawLine(PenBorder, New Point(x:=0, y:=0I), New Point(x:=0, y:=GridSize.Height)) ' Draw the vertical right grid-line. g.DrawLine(PenBorder, New Point(x:=(GridSize.Width - 1I), y:=0I), New Point(x:=(GridSize.Width - 1I), y:=GridSize.Height)) ' Draw the horizontal top grid-line. g.DrawLine(PenBorder, New Point(x:=0I, y:=0I), New Point(x:=GridSize.Width, y:=0I)) ' Draw the horizontal bottom grid-line. g.DrawLine(PenBorder, New Point(x:=0I, y:=(GridSize.Height - 1I)), New Point(x:=GridSize.Width, y:=(GridSize.Height - 1I))) End Using ' PenBorder End If ' DrawBorder End Using ' PenCol End Using ' PenRow End Sub
Y este método lo escribí para obtener los rectangles de la rejilla: ' Get Grid ' By Elektro ' ''' <summary> ''' Calculates the drawing of a grid with the specified size, ''' and returns a<see cref="List(Of Rectangle)"/> that contains the grid-sector specifications. ''' </summary> ''' <param name="GridSize">Indicates the grid size.</param> ''' <param name="Columns">Indicates the amount of columns.</param> ''' <param name="Rows">Indicates the amount of rows.</param> ''' <returns>A <see cref="List(Of Rectangle)"/> that contains the grid-sector specifications.</returns> ''' <exception cref="System.ArgumentException"> ''' GridSize.Width is not divisible by the specified number of columns. ''' or ''' GridSize.Height is not divisible by the specified number of rows. ''' </exception> Private Function GetGrid(ByVal GridSize As Size, ByVal Columns As Integer, ByVal Rows As Integer) As List(Of Rectangle) If Not (GridSize.Width Mod Columns = 0I) Then Throw New ArgumentException( "GridSize.Width is not divisible by the specified number of columns.", "GridSize") Return Nothing ElseIf Not (GridSize.Height Mod Rows = 0I) Then Throw New ArgumentException( "GridSize.Height is not divisible by the specified number of rows.", "GridSize") Return Nothing End If Dim Sectors As New List(Of Rectangle) Dim SectorWidth As Integer = GridSize.Width \ Columns Dim SectorHeight As Integer = GridSize.Height \ Rows For row As Integer = 0I To GridSize.Height - 1 Step (SectorHeight) For col As Integer = 0I To GridSize.Width - 1 Step SectorWidth Sectors.Add(New Rectangle(col, row, SectorWidth, SectorHeight)) Next col Next row Return Sectors End Function
EDITO2:bueno voy a mostrate el resto del código porque total lo hice para ayudarte con el problema y ya no me sirve para nada esto así que antes de tirarlo te lo enseño xD, espero que te sirva. ( esta parte del code lo desarrollé en poco tiempo, está bastante sucio el código y se puede mejorar mucho, pero sirve para hacerse una idea ) Public Property currentsector As Integer = 0 Public Property currentcolor As Color = Color.Gray Public ReadOnly Property GridSectors As List(Of Rectangle) Get Return Me.GetGrid(GridSize:=PictureBox1.ClientRectangle.Size, Columns:=5, Rows:=5) End Get End Property Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Shown Label1.Text = "Sectors: " & GridSectors.Count End Sub Private Sub PictureBox1_Paint(ByVal sender As Object, ByVal e As PaintEventArgs) _ Handles PictureBox1.Paint Me.DrawGrid(g:=e.Graphics, GridSize:=sender.ClientRectangle.Size, Columns:=5, Rows:=5, ColorColumns:=Color.YellowGreen, ColorRows:=Color.YellowGreen, DrawBorder:=True, ColorBorder:=Nothing, ColorStyle:=Drawing2D.DashStyle.Solid) End Sub Private Sub PictureBox1_MouseMove(sender As Object, e As MouseEventArgs) Handles PictureBox1.MouseMove Dim Sectors As List(Of Rectangle) = Me.GridSectors For X As Integer = 0I To (Sectors.Count - 1I) If Sectors(X).Contains(sender.PointToClient(MousePosition)) Then Dim myBrush As New SolidBrush(currentcolor) Using formGraphics As Graphics = sender.CreateGraphics() formGraphics.FillRectangle(myBrush, New Rectangle(Sectors(X).X + 1, Sectors(X).Y + 1, Sectors(X).Width - 2, Sectors(X).Height - 2)) myBrush.Dispose() End Using ' formGraphics currentsector = X + 1 Label2.Text = "Current sector: " & currentsector Else Dim myBrush As New SolidBrush(Color.Black) Using formGraphics As Graphics = sender.CreateGraphics() formGraphics.FillRectangle(myBrush, New Rectangle(Sectors(X).X + 1, Sectors(X).Y + 1, Sectors(X).Width - 2, Sectors(X).Height - 2)) myBrush.Dispose() End Using ' formGraphics End If ' Sectors(X).Contains(...) Next X End Sub Private Sub PictureBox1_Click(sender As Object, e As EventArgs) Handles PictureBox1.MouseDown Label3.Text = "Last Sector Clicked: " & currentsector ' Dim sector As Rectangle = Me.GridSectors(currentsector - 1) currentcolor = Color.Red PictureBox1.Cursor = Cursors.Hand PictureBox1.Update() 'Dim myBrush As New SolidBrush(Color.Red) 'Using formGraphics As Graphics = PictureBox1.CreateGraphics() ' formGraphics.FillRectangle(myBrush, New Rectangle(sector.X + 1, sector.Y + 1, sector.Width - 2, sector.Height - 2)) ' myBrush.Dispose() 'End Using ' formGraphics End Sub Private Sub PictureBox1_Clic(sender As Object, e As EventArgs) Handles PictureBox1.MouseUp Label3.Text = "Last Sector Clicked: " & currentsector ' Dim sector As Rectangle = Me.GridSectors(currentsector - 1) currentcolor = Color.Gray PictureBox1.Cursor = Cursors.Default PictureBox1.Update() 'Dim myBrush As New SolidBrush(Color.Red) 'Using formGraphics As Graphics = PictureBox1.CreateGraphics() ' formGraphics.FillRectangle(myBrush, New Rectangle(sector.X + 1, sector.Y + 1, sector.Width - 2, sector.Height - 2)) ' myBrush.Dispose() 'End Using ' formGraphics End Sub
EDITO1: Fijándome justo la imagen de arriba, al pinchar los pictureBox quiero que aparezcan en tiempo real los resultados en Decimales, Hexadecimales y Binarios.
El tiempo real quiero decir, que desde que pulse un pictureBox, se actualiza los datos dentro de los textBox inficado arriba.
Con usar timer de 0.1 segundo funciona en vez de usar un botón para actualizar.
Espero que se entienda lo que quiero hacer. Esta parte si que no me sale.
Lo siento creo que no te entendí muy bien, ¿que problema tienes actualmente a la hora de actualizar los valores de cada control?. Si vas a utilizar tropecientos pictureboxes entonces deberías suscribirte al evento Click como te explicó seba123neo, y una vez pulses cualquier picturebox actualizaríass los valores (de forma interna) y los imprimirías en los textboxes, ¿cual es el problema? Saludos
|
|
|
6808
|
Programación / .NET (C#, VB.NET, ASP) / Re: Librería de Snippets !! (Compartan aquí sus snippets)
|
en: 20 Agosto 2014, 02:06 am
|
He escrito este ejemplo para mostrar como se puede compartir un espacio de memoria que puede ser leido por diferentes aplicaciones:  Esta sería la aplicación número 1, creen un nuevo proyecto, copien y compilen este Form: ' Example of sharing memory across different running applications. ' By Elektro ' ' ************************* ' This is the Application 1 ' ************************* #Region " Imports " Imports System.IO.MemoryMappedFiles #End Region #Region " Application 2 " ''' <summary> ''' Class MemoryMappedFile_Form1. ''' This should be the Class used to compile our first application. ''' </summary> Public Class MemoryMappedFile_Form1 ' The controls to create on execution-time. Dim WithEvents btMakeFile As New Button ' Writes the memory. Dim WithEvents btReadFile As New Button ' Reads the memory. Dim tbMessage As New TextBox ' Determines the string to map into memory. Dim tbReceptor As New TextBox ' Print the memory read's result. Dim lbInfoButtons As New Label ' Informs the user with a usage hint for the buttons. Dim lbInfotbMessage As New Label ' Informs the user with a usage hint for 'tbMessage'. ''' <summary> ''' Indicates the name of our memory-file. ''' </summary> Private ReadOnly MemoryName As String = "My Memory-File Name" ''' <summary> ''' Indicates the memory buffersize to store the <see cref="MemoryName"/>, in bytes. ''' </summary> Private ReadOnly MemoryBufferSize As Integer = 1024I ''' <summary> ''' Indicates the string to map in memory. ''' </summary> Private ReadOnly Property strMessage As String Get Return tbMessage.Text End Get End Property ''' <summary> ''' Initializes a new instance of the <see cref="MemoryMappedFile_Form1"/> class. ''' </summary> Public Sub New() ' This call is required by the designer. InitializeComponent() ' Set the properties of the controls. With lbInfotbMessage .Location = New Point(20, 10) .Text = "Type in this TextBox the message to write in memory:" .AutoSize = True ' .Size = tbReceptor.Size End With With tbMessage .Text = "Hello world from application one!" .Location = New Point(20, 30) .Size = New Size(310, Me.tbMessage.Height) End With With btMakeFile .Text = "Write Memory" .Size = New Size(130, 45) .Location = New Point(20, 50) End With With btReadFile .Text = "Read Memory" .Size = New Size(130, 45) .Location = New Point(200, 50) End With With tbReceptor .Location = New Point(20, 130) .Size = New Size(310, 100) .Multiline = True End With With lbInfoButtons .Location = New Point(tbReceptor.Location.X, tbReceptor.Location.Y - 30) .Text = "Press '" & btMakeFile.Text & "' button to create the memory file, that memory can be read from both applications." .AutoSize = False .Size = tbReceptor.Size End With ' Set the Form properties. With Me .Text = "Application 1" .Size = New Size(365, 300) .FormBorderStyle = Windows.Forms.FormBorderStyle.FixedSingle .MaximizeBox = False .StartPosition = FormStartPosition.CenterScreen End With ' Add the controls on the UI. Me.Controls.AddRange({lbInfotbMessage, tbMessage, btMakeFile, btReadFile, tbReceptor, lbInfoButtons}) End Sub ''' <summary> ''' Writes a byte sequence into a <see cref="MemoryMappedFile"/>. ''' </summary> ''' <param name="Name">Indicates the name to assign the <see cref="MemoryMappedFile"/>.</param> ''' <param name="BufferLength">Indicates the <see cref="MemoryMappedFile"/> buffer-length to write in.</param> ''' <param name="Data">Indicates the byte-data to write inside the <see cref="MemoryMappedFile"/>.</param> Private Sub MakeMemoryMappedFile(ByVal Name As String, ByVal BufferLength As Integer, ByVal Data As Byte()) ' Create or open the memory-mapped file. Dim MessageFile As MemoryMappedFile = MemoryMappedFile.CreateOrOpen(Name, Me.MemoryBufferSize, MemoryMappedFileAccess.ReadWrite) ' Write the byte-sequence into memory. Using Writer As MemoryMappedViewAccessor = MessageFile.CreateViewAccessor(0L, Me.MemoryBufferSize, MemoryMappedFileAccess.ReadWrite) ' Firstly fill with null all the buffer. Writer.WriteArray(Of Byte)(0L, System.Text.Encoding.ASCII.GetBytes(New String(Nothing, Me.MemoryBufferSize)), 0I, Me.MemoryBufferSize) ' Secondly write the byte-data. Writer.WriteArray(Of Byte)(0L, Data, 0I, Data.Length) End Using ' Writer End Sub ''' <summary> ''' Reads a byte-sequence from a <see cref="MemoryMappedFile"/>. ''' </summary> ''' <param name="Name">Indicates an existing <see cref="MemoryMappedFile"/> assigned name.</param> ''' <param name="BufferLength">The buffer-length to read in.</param> ''' <returns>System.Byte().</returns> Private Function ReadMemoryMappedFile(ByVal Name As String, ByVal BufferLength As Integer) As Byte() Try Using MemoryFile As MemoryMappedFile = MemoryMappedFile.OpenExisting(Name, MemoryMappedFileRights.Read) Using Reader As MemoryMappedViewAccessor = MemoryFile.CreateViewAccessor(0L, BufferLength, MemoryMappedFileAccess.Read) Dim ReadBytes As Byte() = New Byte(BufferLength - 1I) {} Reader.ReadArray(Of Byte)(0L, ReadBytes, 0I, ReadBytes.Length) Return ReadBytes End Using ' Reader End Using ' MemoryFile Catch ex As IO.FileNotFoundException Throw Return Nothing End Try End Function ''' <summary> ''' Handles the 'Click' event of the 'btMakeFile' control. ''' </summary> Private Sub btMakeFile_Click() Handles btMakeFile.Click ' Get the byte-data to create the memory-mapped file. Dim WriteData As Byte() = System.Text.Encoding.ASCII.GetBytes(Me.strMessage) ' Create the memory-mapped file. Me.MakeMemoryMappedFile(Name:=Me.MemoryName, BufferLength:=Me.MemoryBufferSize, Data:=WriteData) End Sub ''' <summary> ''' Handles the 'Click' event of the 'btReadFile' control. ''' </summary> Private Sub btReadFile_Click() Handles btReadFile.Click Dim ReadBytes As Byte() Try ' Read the byte-sequence from memory. ReadBytes = ReadMemoryMappedFile(Name:=Me.MemoryName, BufferLength:=Me.MemoryBufferSize) Catch ex As IO.FileNotFoundException Me.tbReceptor.Text = "Memory-mapped file does not exist." Exit Sub End Try ' Convert the bytes to String. Dim Message As String = System.Text.Encoding.ASCII.GetString(ReadBytes.ToArray) ' Remove null chars (leading zero-bytes) Message = Message.Trim({ControlChars.NullChar}) ' Print the message. tbReceptor.Text = Message End Sub End Class #End Region
Esta sería la aplicación número 2, creen un nuevo proyecto, copien y compilen este Form: ' Example of sharing memory across different running applications. ' By Elektro ' ' ************************* ' This is the Application 2 ' ************************* #Region " Imports " Imports System.IO.MemoryMappedFiles #End Region #Region " Application 2 " ''' <summary> ''' Class MemoryMappedFile_Form2. ''' This should be the Class used to compile our first application. ''' </summary> Public Class MemoryMappedFile_Form2 ' The controls to create on execution-time. Dim WithEvents btMakeFile As New Button ' Writes the memory. Dim WithEvents btReadFile As New Button ' Reads the memory. Dim tbMessage As New TextBox ' Determines the string to map into memory. Dim tbReceptor As New TextBox ' Print the memory read's result. Dim lbInfoButtons As New Label ' Informs the user with a usage hint for the buttons. Dim lbInfotbMessage As New Label ' Informs the user with a usage hint for 'tbMessage'. ''' <summary> ''' Indicates the name of our memory-file. ''' </summary> Private ReadOnly MemoryName As String = "My Memory-File Name" ''' <summary> ''' Indicates the memory buffersize to store the <see cref="MemoryName"/>, in bytes. ''' </summary> Private ReadOnly MemoryBufferSize As Integer = 1024I ''' <summary> ''' Indicates the string to map in memory. ''' </summary> Private ReadOnly Property strMessage As String Get Return tbMessage.Text End Get End Property ''' <summary> ''' Initializes a new instance of the <see cref="MemoryMappedFile_Form2"/> class. ''' </summary> Public Sub New() ' This call is required by the designer. InitializeComponent() ' Set the properties of the controls. With lbInfotbMessage .Location = New Point(20, 10) .Text = "Type in this TextBox the message to write in memory:" .AutoSize = True ' .Size = tbReceptor.Size End With With tbMessage .Text = "Hello world from application two!" .Location = New Point(20, 30) .Size = New Size(310, Me.tbMessage.Height) End With With btMakeFile .Text = "Write Memory" .Size = New Size(130, 45) .Location = New Point(20, 50) End With With btReadFile .Text = "Read Memory" .Size = New Size(130, 45) .Location = New Point(200, 50) End With With tbReceptor .Location = New Point(20, 130) .Size = New Size(310, 100) .Multiline = True End With With lbInfoButtons .Location = New Point(tbReceptor.Location.X, tbReceptor.Location.Y - 30) .Text = "Press '" & btMakeFile.Text & "' button to create the memory file, that memory can be read from both applications." .AutoSize = False .Size = tbReceptor.Size End With ' Set the Form properties. With Me .Text = "Application 2" .Size = New Size(365, 300) .FormBorderStyle = Windows.Forms.FormBorderStyle.FixedSingle .MaximizeBox = False .StartPosition = FormStartPosition.CenterScreen End With ' Add the controls on the UI. Me.Controls.AddRange({lbInfotbMessage, tbMessage, btMakeFile, btReadFile, tbReceptor, lbInfoButtons}) End Sub ''' <summary> ''' Writes a byte sequence into a <see cref="MemoryMappedFile"/>. ''' </summary> ''' <param name="Name">Indicates the name to assign the <see cref="MemoryMappedFile"/>.</param> ''' <param name="BufferLength">Indicates the <see cref="MemoryMappedFile"/> buffer-length to write in.</param> ''' <param name="Data">Indicates the byte-data to write inside the <see cref="MemoryMappedFile"/>.</param> Private Sub MakeMemoryMappedFile(ByVal Name As String, ByVal BufferLength As Integer, ByVal Data As Byte()) ' Create or open the memory-mapped file. Dim MessageFile As MemoryMappedFile = MemoryMappedFile.CreateOrOpen(Name, Me.MemoryBufferSize, MemoryMappedFileAccess.ReadWrite) ' Write the byte-sequence into memory. Using Writer As MemoryMappedViewAccessor = MessageFile.CreateViewAccessor(0L, Me.MemoryBufferSize, MemoryMappedFileAccess.ReadWrite) ' Firstly fill with null all the buffer. Writer.WriteArray(Of Byte)(0L, System.Text.Encoding.ASCII.GetBytes(New String(Nothing, Me.MemoryBufferSize)), 0I, Me.MemoryBufferSize) ' Secondly write the byte-data. Writer.WriteArray(Of Byte)(0L, Data, 0I, Data.Length) End Using ' Writer End Sub ''' <summary> ''' Reads a byte-sequence from a <see cref="MemoryMappedFile"/>. ''' </summary> ''' <param name="Name">Indicates an existing <see cref="MemoryMappedFile"/> assigned name.</param> ''' <param name="BufferLength">The buffer-length to read in.</param> ''' <returns>System.Byte().</returns> Private Function ReadMemoryMappedFile(ByVal Name As String, ByVal BufferLength As Integer) As Byte() Try Using MemoryFile As MemoryMappedFile = MemoryMappedFile.OpenExisting(Name, MemoryMappedFileRights.Read) Using Reader As MemoryMappedViewAccessor = MemoryFile.CreateViewAccessor(0L, BufferLength, MemoryMappedFileAccess.Read) Dim ReadBytes As Byte() = New Byte(BufferLength - 1I) {} Reader.ReadArray(Of Byte)(0L, ReadBytes, 0I, ReadBytes.Length) Return ReadBytes End Using ' Reader End Using ' MemoryFile Catch ex As IO.FileNotFoundException Throw Return Nothing End Try End Function ''' <summary> ''' Handles the 'Click' event of the 'btMakeFile' control. ''' </summary> Private Sub btMakeFile_Click() Handles btMakeFile.Click ' Get the byte-data to create the memory-mapped file. Dim WriteData As Byte() = System.Text.Encoding.ASCII.GetBytes(Me.strMessage) ' Create the memory-mapped file. Me.MakeMemoryMappedFile(Name:=Me.MemoryName, BufferLength:=Me.MemoryBufferSize, Data:=WriteData) End Sub ''' <summary> ''' Handles the 'Click' event of the 'btReadFile' control. ''' </summary> Private Sub btReadFile_Click() Handles btReadFile.Click Dim ReadBytes As Byte() Try ' Read the byte-sequence from memory. ReadBytes = ReadMemoryMappedFile(Name:=Me.MemoryName, BufferLength:=Me.MemoryBufferSize) Catch ex As IO.FileNotFoundException Me.tbReceptor.Text = "Memory-mapped file does not exist." Exit Sub End Try ' Convert the bytes to String. Dim Message As String = System.Text.Encoding.ASCII.GetString(ReadBytes.ToArray) ' Remove null chars (leading zero-bytes) Message = Message.Trim({ControlChars.NullChar}) ' Print the message. tbReceptor.Text = Message End Sub End Class #End Region
Ahora ya solo tienen que ejecutar ambas aplicaciones para testear. Saludos!
|
|
|
6809
|
Programación / .NET (C#, VB.NET, ASP) / Re: Librería de Snippets !! (Compartan aquí sus snippets)
|
en: 19 Agosto 2014, 22:06 pm
|
Un ejemplo de uso de la librería MagicGraphics: http://www.codeproject.com/Articles/19188/Magic-Graphics 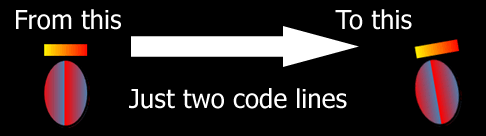
Escribí este Form para jugar un poco con la funcionalidad de esta librería, la verdad es que es muy sencillo.  Public Class MagicGraphics_Test Private WithEvents RotationTimer As New Timer With {.Enabled = True, .Interval = 25} Dim SC As MagicGraphics.ShapeContainer Private Sub Tst_Shown() Handles MyBase.Shown SC = New MagicGraphics.ShapeContainer(PictureBox1.CreateGraphics, PictureBox1.Width, PictureBox1.Height, Color.Black, PictureBox1.Image) PictureBox1.Image = SC.BMP SC.AutoFlush = False Dim Sq As New MagicGraphics.Rectangle(New Pen(Color.Black, 3), Brushes.Aqua, 60, 20, 50, 50) Sq.FillingBrush = New Drawing2D.LinearGradientBrush(New Point(0, 0), New Point(60, 0), Color.Yellow, Color.Red) SC.AddShape(Sq) Dim El As New MagicGraphics.Ellipse(New Pen(Color.Black, 3), Brushes.Olive, 60, 88, 50, 71) El.FillingBrush = New Drawing2D.LinearGradientBrush(New Point(0, 0), New Point(30, 0), Color.Red, Color.SteelBlue) SC.AddShape(El) RotationTimer.Start() End Sub Private Sub RotationTimer_Tick() Handles RotationTimer.Tick Static Direction As Integer = 1I ' 0 = Left, 1 = Right For X As Integer = 0I To (SC.ShapesL.Count - 1) Dim shp As MagicGraphics.Shape = SC.ShapesL(X) shp.Rotate(-8) If shp.Location.X > (PictureBox1.Width - shp.Width) Then Direction = 1I ' Right ElseIf shp.Location.X < PictureBox1.Location.X Then Direction = 0I ' Left End If If Direction = 0 Then shp.Move(shp.Location.X + 2, shp.Location.Y) Else shp.Move(shp.Location.X - 2, shp.Location.Y) End If ' Debug.WriteLine(String.Format("Shape {0} Rotation: {1}", CStr(X), shp.Rotation)) Next X SC.Flush() End Sub End Class
|
|
|
6810
|
Programación / .NET (C#, VB.NET, ASP) / Re: Mis malas combinaciones :(
|
en: 19 Agosto 2014, 13:49 pm
|
Mil gracias de nuevo ahora si pude hacerlo funcionar Bien aca donde me pones esto , es lo debería de cambiar por mi variable " result " que es la que tiene los números después de efectuada la operación que hago en mi programa ? ReadOnly FixedValues As Integer() = {1, 5, 19, 22, 34, 55, 66, 88, 99, etc...}
¿A cual de las miles de variables que bautizaste con el nombre de "Result" te refieres?  Supongo que si, en la variable FixedValues debes especificarle los numeros que se tomarán para hacer las combinaciones, en tu ejemplo pusiste del 1 al 30, no se si harás eso con la variable "result" que mencionas, pero creo que ya te hiciste una idea de lo que debe ir en esa variable FixedValues (valores fijos). por lo demas funciona como queria Me alegro de oir esoPD: Por si acaso te recuerdo que para ordenar de mayor a menor lo tienes facil usando una LINQ-query: dim values as integer() = (from n as integer in TUSNUMEROS order by n ascending).toarray
o el método Sort, en una lista. Saludos!
|
|
|
|
|
|
|